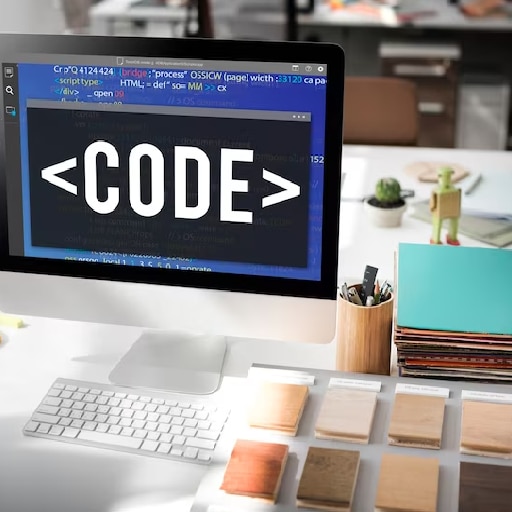
Node.js and MongoDB are two powerful technologies that can be used together to build full-stack JavaScript applications. In this tutorial, we will walk through the process of building a simple application using these technologies.
Prerequisites:
– Basic knowledge of JavaScript
– Node.js and npm installed on your machine
– MongoDB installed on your machine
Step 1: Set up the project
1. Create a new directory for your project and navigate to it in your terminal.
2. Initialize a new Node.js project by running the following command:
“`
npm init -y
“`
3. Install the necessary dependencies by running the following command:
“`
npm install express mongoose
“`
Step 2: Create the server
1. Create a new file called `server.js` in your project directory.
2. Import the necessary modules at the top of the file:
“`javascript
const express = require(‘express’);
const mongoose = require(‘mongoose’);
“`
3. Create an instance of the Express application:
“`javascript
const app = express();
“`
4. Set up the server to listen on a specific port:
“`javascript
const port = 3000;
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
“`
Step 3: Connect to MongoDB
1. Create a new file called `db.js` in your project directory.
2. Import the necessary modules at the top of the file:
“`javascript
const mongoose = require(‘mongoose’);
“`
3. Connect to the MongoDB database:
“`javascript
mongoose.connect(‘mongodb://localhost/myapp’, {
useNewUrlParser: true,
useUnifiedTopology: true
})
.then(() => {
console.log(‘Connected to MongoDB’);
})
.catch((error) => {
console.error(‘Error connecting to MongoDB’, error);
});
“`
4. Import the `db.js` file in your `server.js` file:
“`javascript
require(‘./db’);
“`
Step 4: Create a model
1. Create a new file called `model.js` in your project directory.
2. Import the necessary modules at the top of the file:
“`javascript
const mongoose = require(‘mongoose’);
“`
3. Define a schema for your data:
“`javascript
const schema = new mongoose.Schema({
name: String,
age: Number
});
“`
4. Create a model based on the schema:
“`javascript
const Model = mongoose.model(‘Model’, schema);
“`
5. Export the model:
“`javascript
module.exports = Model;
“`
Step 5: Create routes
1. Import the necessary modules at the top of your `server.js` file:
“`javascript
const Model = require(‘./model’);
“`
2. Create a route to get all the data:
“`javascript
app.get(‘/data’, (req, res) => {
Model.find()
.then((data) => {
res.json(data);
})
.catch((error) => {
console.error(‘Error getting data’, error);
res.status(500).json({ error: ‘Error getting data’ });
});
});
“`
3. Create a route to create new data:
“`javascript
app.post(‘/data’, (req, res) => {
const { name, age } = req.body;
const model = new Model({ name, age });
model.save()
.then(() => {
res.json({ message: ‘Data created successfully’ });
})
.catch((error) => {
console.error(‘Error creating data’, error);
res.status(500).json({ error: ‘Error creating data’ });
});
});
“`
Step 6: Test the application
1. Start the server by running the following command in your terminal:
“`
node server.js
“`
2. Open your browser and navigate to `http://localhost:3000/data`. You should see an empty array.
3. Use a tool like Postman to send a POST request to `http://localhost:3000/data` with a JSON body containing `name` and `age` properties.
4. Refresh the page at `http://localhost:3000/data` and you should see the data you just created.
Congratulations! You have successfully built a full-stack JavaScript application using Node.js and MongoDB. This is just a basic example, but you can expand on it by adding more routes and functionality to suit your needs.