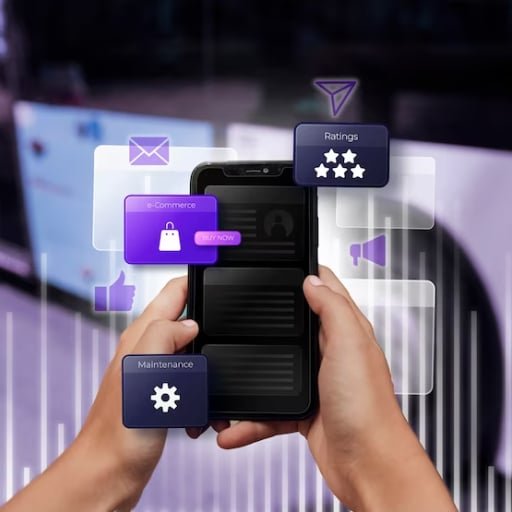
To create a chat application with Ruby on Rails, you can follow these steps:
1. Set up a new Rails application by running the following command in your terminal:
“`
rails new chat_app
“`
2. Change into the newly created application directory:
“`
cd chat_app
“`
3. Generate a model for the chat messages:
“`
rails generate model Message content:text
“`
4. Run the database migration to create the messages table:
“`
rails db:migrate
“`
5. Generate a controller for the chat messages:
“`
rails generate controller Messages
“`
6. Open the `app/controllers/messages_controller.rb` file and add the following actions:
“`ruby
class MessagesController < ApplicationController
def index
@messages = Message.all
end
def create
@message = Message.new(message_params)
if @message.save
ActionCable.server.broadcast ‘messages_channel’, message: render_message(@message)
end
end
private
def message_params
params.require(:message).permit(:content)
end
def render_message(message)
ApplicationController.render(partial: ‘messages/message’, locals: { message: message })
end
end
“`
7. Create a new file `app/views/messages/index.html.erb` and add the following code:
“`html
Chat Messages
<%= render @messages %>
<%= form_with(model: Message.new, url: messages_path, remote: true) do |form| %>
<%= form.text_area :content %>
<%= form.submit ‘Send’ %>
<% end %>
“`
8. Create a new file `app/views/messages/_message.html.erb` and add the following code:
“`html
<%= message.content %>
“`
9. Open the `config/routes.rb` file and add the following route:
“`ruby
Rails.application.routes.draw do
resources :messages
root ‘messages#index’
end
“`
10. Generate a channel for real-time updates using Action Cable:
“`
rails generate channel Messages
“`
11. Open the `app/channels/messages_channel.rb` file and add the following code:
“`ruby
class MessagesChannel < ApplicationCable::Channel
def subscribed
stream_from ‘messages_channel’
end
end
“`
12. Open the `app/assets/javascripts/channels/messages.coffee` file and add the following code:
“`coffee
App.messages = App.cable.subscriptions.create("MessagesChannel", {
received: (data) ->
$(‘#messages’).append(data.message)
});
“`
13. Start the Rails server:
“`
rails server
“`
Now, you should be able to access the chat application in your browser at `http://localhost:3000`. Multiple users can send messages, and they will be displayed in real-time for all connected users.