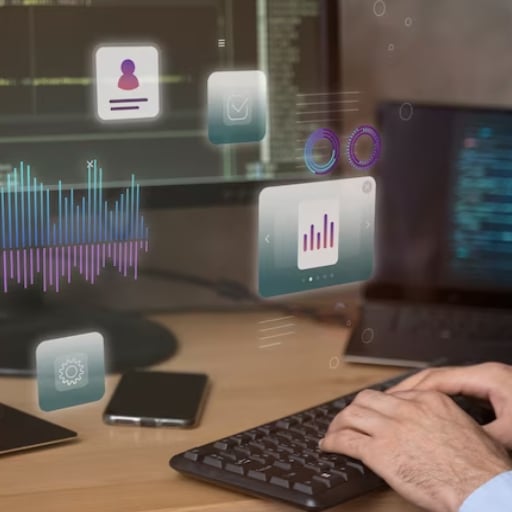
To work with MySQL database in PHP, you need to establish a connection, execute queries, and fetch results. Here’s a step-by-step guide on how to achieve this:
1. Establish a Connection:
– Use the `mysqli_connect()` function to connect to the MySQL database. It takes four parameters: hostname, username, password, and database name.
– Example: `$conn = mysqli_connect("localhost", "username", "password", "database_name");`
2. Execute Queries:
– Use the `mysqli_query()` function to execute SQL queries. It takes two parameters: the connection variable and the SQL query.
– Example: `$result = mysqli_query($conn, "SELECT * FROM table_name");`
3. Fetch Results:
– Use the `mysqli_fetch_assoc()` function to fetch the result set as an associative array. It takes the result variable as a parameter.
– Example: `while ($row = mysqli_fetch_assoc($result)) { echo $row[‘column_name’]; }`
4. Close the Connection:
– Use the `mysqli_close()` function to close the database connection when you’re done.
– Example: `mysqli_close($conn);`
Here’s a complete example that demonstrates the above steps:
“`php
// Step 1: Establish a Connection
$conn = mysqli_connect("localhost", "username", "password", "database_name");
// Step 2: Execute Queries
$result = mysqli_query($conn, "SELECT * FROM table_name");
// Step 3: Fetch Results
while ($row = mysqli_fetch_assoc($result)) {
echo $row[‘column_name’];
}
// Step 4: Close the Connection
mysqli_close($conn);
?>
“`
Note: Replace "localhost", "username", "password", "database_name", and "table_name" with your actual values.