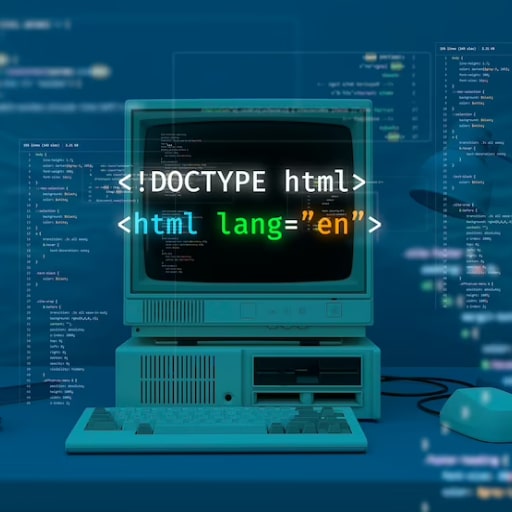
Sure! Here is a step-by-step tutorial on how to dockerize an Express.js application:
Step 1: Install Docker
First, make sure you have Docker installed on your machine. You can download and install Docker from the official website (https://www.docker.com/get-started).
Step 2: Create a new directory for your project
Create a new directory for your Express.js application. Open a terminal and navigate to the directory.
Step 3: Initialize a new Node.js project
Run the following command to initialize a new Node.js project in the directory:
“`
npm init -y
“`
Step 4: Install Express.js
Install Express.js as a dependency for your project by running the following command:
“`
npm install express
“`
Step 5: Create an Express.js application
Create a new file named `app.js` in the project directory and add the following code to create a basic Express.js application:
“`javascript
const express = require(‘express’);
const app = express();
app.get(‘/’, (req, res) => {
res.send(‘Hello, Docker!’);
});
app.listen(3000, () => {
console.log(‘Server is running on port 3000’);
});
“`
Step 6: Create a Dockerfile
Create a new file named `Dockerfile` in the project directory and add the following code:
“`
# Use the official Node.js image as the base image
FROM node:14
# Set the working directory in the container
WORKDIR /app
# Copy package.json and package-lock.json to the working directory
COPY package*.json ./
# Install project dependencies
RUN npm install
# Copy the rest of the application code to the working directory
COPY . .
# Expose port 3000
EXPOSE 3000
# Start the application
CMD [ "node", "app.js" ]
“`
Step 7: Build the Docker image
Open a terminal and navigate to the project directory. Run the following command to build the Docker image:
“`
docker build -t express-app .
“`
Step 8: Run the Docker container
Once the Docker image is built, you can run a container using the following command:
“`
docker run -p 3000:3000 express-app
“`
Step 9: Test the application
Open a web browser and navigate to `http://localhost:3000`. You should see the message "Hello, Docker!" displayed.
That’s it! You have successfully dockerized your Express.js application. You can now distribute and run your application in any environment that has Docker installed.