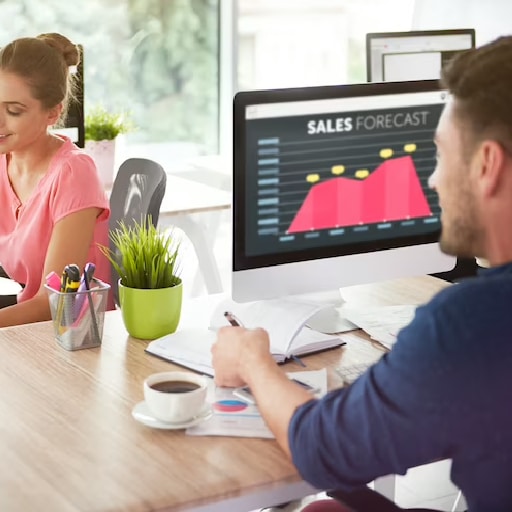
Creating a blogging platform with Node.js and Express can be a great way to showcase your web development skills and provide a platform for users to share their thoughts and ideas. In this guide, we will walk through the steps to set up a basic blogging platform using Node.js and Express.
Step 1: Set up the project
1. Create a new directory for your project and navigate to it in your terminal.
2. Initialize a new Node.js project by running `npm init` and following the prompts.
3. Install Express by running `npm install express`.
4. Create a new file called `index.js` and open it in your preferred code editor.
Step 2: Set up the Express server
1. In `index.js`, require the Express module and create a new instance of the Express application.
“`javascript
const express = require(‘express’);
const app = express();
“`
2. Set up the server to listen on a specific port (e.g., 3000) by adding the following code:
“`javascript
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
“`
Step 3: Set up the routes
1. Create a new directory called `routes` in your project directory.
2. Inside the `routes` directory, create a new file called `blog.js` and open it in your code editor.
3. In `blog.js`, require the Express module and create a new instance of the Express Router.
“`javascript
const express = require(‘express’);
const router = express.Router();
“`
4. Define the routes for your blogging platform. For example, you can have routes for creating a new blog post, viewing a specific blog post, and viewing all blog posts.
“`javascript
// Create a new blog post
router.post(‘/posts’, (req, res) => {
// Logic to create a new blog post
});
// View a specific blog post
router.get(‘/posts/:id’, (req, res) => {
// Logic to retrieve and display a specific blog post
});
// View all blog posts
router.get(‘/posts’, (req, res) => {
// Logic to retrieve and display all blog posts
});
“`
5. Export the router so that it can be used in the main `index.js` file.
“`javascript
module.exports = router;
“`
6. In `index.js`, require the `blog.js` file and use the router in your Express application.
“`javascript
const blogRouter = require(‘./routes/blog’);
app.use(‘/blog’, blogRouter);
“`
Step 4: Set up the views
1. Create a new directory called `views` in your project directory.
2. Inside the `views` directory, create a new file called `index.ejs` and open it in your code editor.
3. Add the HTML and EJS code for your blog’s homepage. For example, you can have a form for creating a new blog post and a list of all existing blog posts.
“`html
Welcome to My Blog
Create a New Blog Post
All Blog Posts
- <%= post.title %>
<% posts.forEach((post) => { %>
<% }); %>
“`
4. In `blog.js`, require the `path` module and set up a route to render the `index.ejs` view.
“`javascript
const path = require(‘path’);
// View all blog posts
router.get(‘/posts’, (req, res) => {
const posts = []; // Replace with logic to retrieve all blog posts
res.render(path.join(__dirname, ‘../views/index.ejs’), { posts });
});
“`
Step 5: Run the application
1. In your terminal, run `node index.js` to start the Express server.
2. Open your web browser and navigate to `http://localhost:3000/blog/posts` to view the blogging platform.
Congratulations! You have created a basic blogging platform using Node.js and Express. From here, you can continue to enhance and expand the functionality of your platform by adding features such as user authentication, comments, and categories.