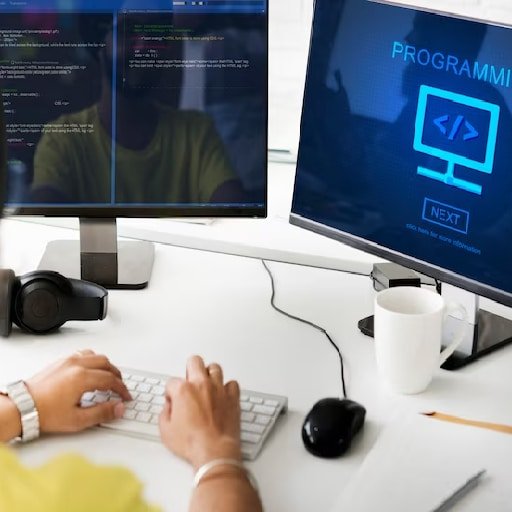
Building scalable web applications with Node.js involves using various techniques and tools to ensure that the application can handle a large number of concurrent users and requests without sacrificing performance. Here are some key considerations and best practices for building scalable web applications with Node.js:
1. Use asynchronous programming: Node.js is built on an event-driven, non-blocking I/O model, which makes it well-suited for handling concurrent requests. Use asynchronous programming techniques, such as callbacks, promises, or async/await, to avoid blocking the event loop and maximize the application’s throughput.
2. Employ clustering: Node.js provides a built-in module called "cluster" that allows you to create multiple worker processes, each running on a separate CPU core. Clustering helps distribute the load across multiple cores and enables your application to handle more concurrent requests.
3. Utilize load balancing: Load balancing is essential for distributing incoming requests across multiple instances of your application. You can use a load balancer like Nginx or HAProxy to evenly distribute the traffic among multiple Node.js instances or use a cloud-based load balancing service like AWS Elastic Load Balancer.
4. Cache frequently accessed data: Caching can significantly improve the performance and scalability of your application. Use a caching layer, such as Redis or Memcached, to store frequently accessed data in memory and reduce the load on your database or other external services.
5. Optimize database queries: Database queries can be a common bottleneck in web applications. Use techniques like indexing, denormalization, and query optimization to ensure that your database queries are efficient and performant. Consider using a NoSQL database like MongoDB or a caching layer like Redis for faster data retrieval.
6. Use a message queue: Asynchronous message queues, such as RabbitMQ or Apache Kafka, can help decouple different parts of your application and improve scalability. Use message queues to offload time-consuming tasks or to handle inter-process communication between different components of your application.
7. Monitor and scale dynamically: Implement monitoring and logging tools to gain insights into your application’s performance and identify potential bottlenecks. Use tools like New Relic, Datadog, or Prometheus to monitor key metrics and set up alerts for abnormal behavior. Additionally, use auto-scaling features provided by cloud platforms like AWS or Google Cloud to automatically scale your application based on demand.
8. Use a microservices architecture: Breaking your application into smaller, loosely coupled services can improve scalability and maintainability. Each microservice can be developed and deployed independently, allowing you to scale individual components as needed.
9. Implement horizontal scaling: Horizontal scaling involves adding more servers or instances to your application to handle increased traffic. Use containerization technologies like Docker and orchestration tools like Kubernetes to easily scale your application horizontally.
10. Perform load testing: Regularly perform load testing to simulate high traffic scenarios and identify any performance bottlenecks. Tools like Apache JMeter or LoadRunner can help you simulate concurrent users and measure the performance of your application under different loads.
By following these best practices and leveraging the scalability features of Node.js, you can build web applications that can handle a large number of concurrent users and requests while maintaining optimal performance.