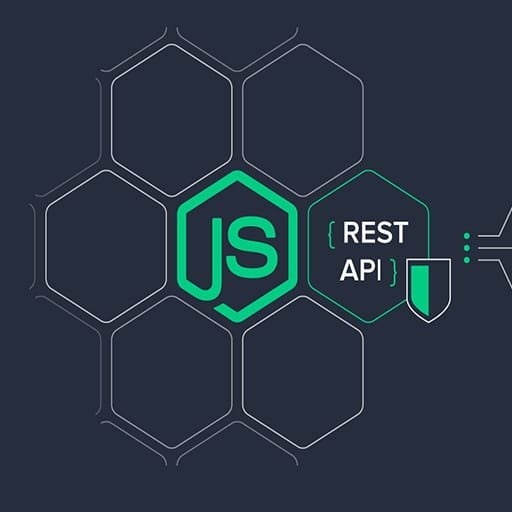
To create RESTful APIs with Node.js and Express, follow these steps:
1. Set up a new Node.js project by creating a new directory and running `npm init` to initialize a new `package.json` file.
2. Install Express by running `npm install express`.
3. Create a new file, such as `server.js`, and require Express:
“`javascript
const express = require(‘express’);
const app = express();
“`
4. Define your API routes using Express’s routing methods (`app.get`, `app.post`, `app.put`, `app.delete`, etc.). For example:
“`javascript
app.get(‘/api/users’, (req, res) => {
// Logic to fetch all users from a database or any other data source
res.json(users);
});
app.post(‘/api/users’, (req, res) => {
// Logic to create a new user
res.json(newUser);
});
app.put(‘/api/users/:id’, (req, res) => {
// Logic to update a user with the given ID
res.json(updatedUser);
});
app.delete(‘/api/users/:id’, (req, res) => {
// Logic to delete a user with the given ID
res.json(deletedUser);
});
“`
5. Start the server by listening on a specific port:
“`javascript
const port = 3000;
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
“`
6. Run the server by executing `node server.js` in the terminal.
Now you have a basic setup for creating RESTful APIs with Node.js and Express. You can add more routes and logic as needed for your specific application.