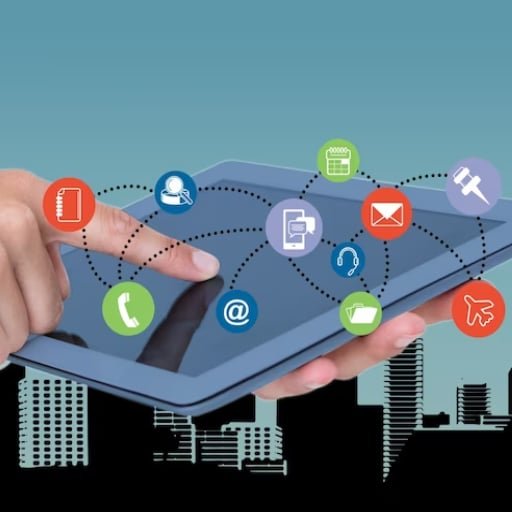
Ruby on Rails is a popular web development framework that allows developers to build robust and scalable applications quickly. In this guide, we will explore how to build real-time chat applications using Ruby on Rails.
To get started, make sure you have Ruby and Rails installed on your machine. You can check your Ruby version by running `ruby -v` in your terminal, and your Rails version by running `rails -v`.
1. Set up a new Rails application:
“`
rails new chat_app
cd chat_app
“`
2. Generate a new model for storing messages:
“`
rails generate model Message content:text
rails db:migrate
“`
3. Create a new controller for handling chat functionality:
“`
rails generate controller Chat
“`
4. In the `app/controllers/chat_controller.rb` file, add the following methods:
“`ruby
class ChatController < ApplicationController
def index
@messages = Message.all
end
def create
@message = Message.create(content: params[:content])
ActionCable.server.broadcast ‘chat_channel’, message: @message.content
end
end
“`
5. In the `app/views/chat/index.html.erb` file, add the following code to display the chat messages:
“`html
Chat
<% @messages.each do |message| %>
<%= message.content %>
<% end %>
<%= form_with url: chat_create_path, method: :post, remote: true do |form| %>
<%= form.text_field :content %>
<%= form.submit ‘Send’ %>
<% end %>
“`
6. Create a new channel for handling real-time updates:
“`
rails generate channel Chat
“`
7. In the `app/channels/chat_channel.rb` file, add the following code to handle real-time updates:
“`ruby
class ChatChannel < ApplicationCable::Channel
def subscribed
stream_from ‘chat_channel’
end
end
“`
8. In the `app/assets/javascripts/channels/chat.coffee` file, add the following code to handle real-time updates on the client-side:
“`coffee
App.chat = App.cable.subscriptions.create "ChatChannel",
received: (data) ->
$(‘#messages’).append(‘
’ + data.message + ‘
’)“`
9. Start the Rails server:
“`
rails server
“`
Now, you should be able to access the chat application at `http://localhost:3000/chat`. You can open multiple browser windows and see the chat messages being updated in real-time.
This is a basic example of building a real-time chat application using Ruby on Rails. You can further enhance the application by adding features like user authentication, private messaging, and message persistence.