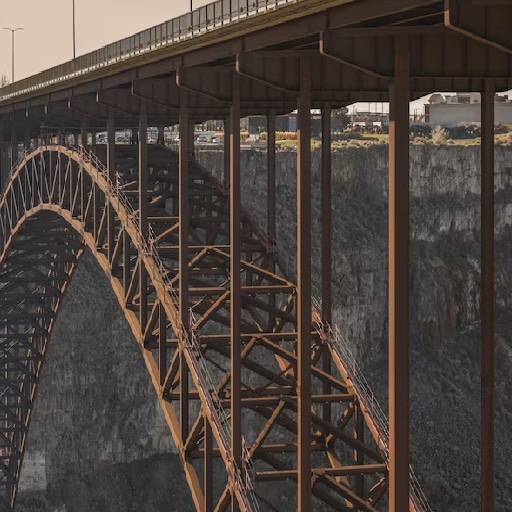
To build a job board with Ruby on Rails, you can follow these steps:
1. Set up a new Rails application by running the following command in your terminal:
“`
rails new job_board
“`
2. Change into the project directory:
“`
cd job_board
“`
3. Generate a scaffold for the job model, which will create the necessary files and database migration for the job board:
“`
rails generate scaffold Job title:string description:text company:string location:string
“`
4. Run the database migration to create the jobs table in the database:
“`
rails db:migrate
“`
5. Open the `app/models/job.rb` file and add any validations or associations you need for the job model. For example, you can add a validation to ensure that the title and description are present:
“`ruby
class Job < ApplicationRecord
validates :title, :description, presence: true
end
“`
6. Open the `config/routes.rb` file and add a route for the jobs resource:
“`ruby
Rails.application.routes.draw do
resources :jobs
root ‘jobs#index’
end
“`
7. Open the `app/controllers/jobs_controller.rb` file and update the controller actions as needed. For example, you can add a `new` action to display a form for creating a new job:
“`ruby
class JobsController < ApplicationController
def new
@job = Job.new
end
def create
@job = Job.new(job_params)
if @job.save
redirect_to @job, notice: ‘Job was successfully created.’
else
render :new
end
end
private
def job_params
params.require(:job).permit(:title, :description, :company, :location)
end
end
“`
8. Create the necessary views in the `app/views/jobs` directory. For example, you can create a `new.html.erb` file to display the job creation form:
“`erb
New Job
<%= render ‘form’, job: @job %>
<%= link_to ‘Back’, jobs_path %>
“`
9. Create a partial view `_form.html.erb` to render the job form:
“`erb
<%= form_with(model: job, local: true) do |form| %>
<% if job.errors.any? %>
<%= pluralize(job.errors.count, "error") %> prohibited this job from being saved:
- <%= message %>
<% job.errors.full_messages.each do |message| %>
<% end %>
<% end %>
<%= form.label :title %>
<%= form.text_field :title %>
<%= form.label :description %>
<%= form.text_area :description %>
<%= form.label :company %>
<%= form.text_field :company %>
<%= form.label :location %>
<%= form.text_field :location %>
<%= form.submit %>
<% end %>
“`
10. Customize the views and add any additional features you need for your job board, such as editing or deleting jobs.
11. Start the Rails server to see your job board in action:
“`
rails server
“`
You can now access your job board by visiting `http://localhost:3000/jobs` in your web browser.