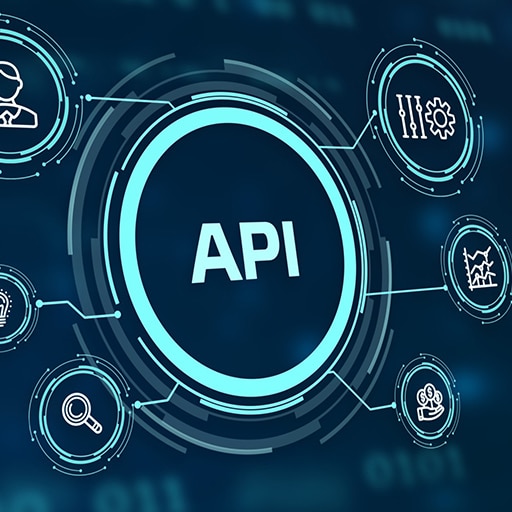
Working with APIs in PHP can be a powerful way to integrate external services and data into your web applications. In this comprehensive guide, we will cover the basics of working with APIs in PHP, including how to make API requests, handle responses, and authenticate with APIs.
1. What is an API?
An API (Application Programming Interface) is a set of rules and protocols that allows different software applications to communicate with each other. APIs enable developers to access and use the functionality of external services or platforms, such as social media platforms, payment gateways, or weather services.
2. Making API Requests
To interact with an API in PHP, you need to make HTTP requests to the API endpoints. PHP provides several ways to make HTTP requests, including the built-in `file_get_contents()` function, the cURL library, and various HTTP client libraries like Guzzle.
3. Handling API Responses
Once you make an API request, you will receive a response from the API server. The response can be in various formats, such as JSON, XML, or plain text. In PHP, you can use built-in functions like `json_decode()` or libraries like Symfony’s Serializer component to parse and handle the API response.
4. Authenticating with APIs
Many APIs require authentication to ensure that only authorized users can access their resources. There are different authentication methods used by APIs, such as API keys, OAuth, or token-based authentication. In PHP, you can include authentication credentials in the API request headers or use libraries like OAuth2-client to handle more complex authentication flows.
5. Error Handling and Rate Limiting
APIs can return errors or impose rate limits to prevent abuse. It’s important to handle API errors gracefully and implement rate limiting strategies in your PHP code. You can use try-catch blocks to catch and handle API exceptions, and implement rate limiting logic to avoid exceeding API usage limits.
6. API Documentation and Testing
API documentation is crucial for understanding how to use an API correctly. Most APIs provide detailed documentation that explains the available endpoints, request parameters, and response formats. Additionally, you can use tools like Postman or Insomnia to test API requests and verify their responses before integrating them into your PHP code.
7. Caching API Responses
To improve performance and reduce API usage, you can implement caching mechanisms for API responses. PHP provides various caching options, such as using the `memcached` or `redis` extensions, or using libraries like Symfony’s Cache component. Caching can help reduce the number of API requests and speed up your application.
8. Handling Pagination and Filtering
Some APIs return large amounts of data that need to be paginated or filtered. Pagination allows you to retrieve data in smaller chunks, while filtering enables you to request specific subsets of data. You can use query parameters in your API requests to specify the page number, page size, or filtering criteria.
9. Monitoring and Logging
When working with APIs, it’s important to monitor their usage and log any errors or issues. You can use tools like New Relic or Datadog to monitor API performance and set up alerts for critical issues. Additionally, logging API requests and responses can help with debugging and troubleshooting.
In conclusion, working with APIs in PHP opens up a world of possibilities for integrating external services and data into your applications. By following this comprehensive guide, you will have a solid foundation for working with APIs and building powerful, data-driven PHP applications.