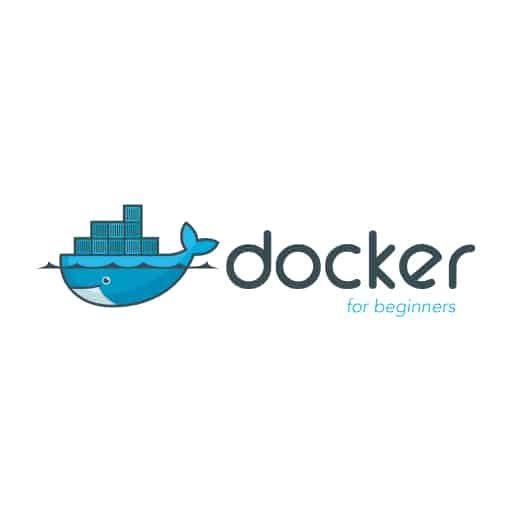
Docker is an open-source platform that allows you to automate the deployment, scaling, and management of applications using containerization. Containers are lightweight, isolated environments that package everything needed to run an application, including the code, runtime, system tools, and libraries.
If you’re new to Docker and want to get started, here’s a beginner’s guide to help you understand the basics and start using Docker for your projects.
1. Install Docker:
– Docker is available for various operating systems, including Windows, macOS, and Linux. Visit the Docker website (https://www.docker.com/) and download the appropriate version for your system.
– Follow the installation instructions provided by Docker to complete the installation process.
2. Verify Docker installation:
– Open a terminal or command prompt and run the following command to verify that Docker is installed correctly:
“`
docker version
“`
– This command will display the Docker version information if the installation was successful.
3. Run your first container:
– Docker images are the building blocks of containers. You can think of an image as a template for creating containers.
– Run the following command to download and run a simple "Hello World" container:
“`
docker run hello-world
“`
– Docker will download the "hello-world" image from the Docker Hub registry and run it as a container. You should see a message indicating that Docker is working correctly.
4. Explore Docker commands:
– Docker provides a command-line interface (CLI) with various commands to manage containers, images, networks, and volumes.
– Some commonly used commands include:
– `docker ps`: List running containers.
– `docker images`: List available images.
– `docker pull
– `docker build -t
– `docker stop
– `docker rm
– Run `docker –help` or `docker
5. Create your own Dockerfile:
– Dockerfiles are text files that contain instructions for building Docker images.
– Create a new file named `Dockerfile` in your project directory and define the steps to build your image.
– For example, a simple Dockerfile for a Node.js application could look like this:
“`
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
CMD ["npm", "start"]
“`
– This Dockerfile specifies a base image, sets the working directory, copies the package.json file, installs dependencies, copies the application code, and defines the command to run the application.
– Build the image using the following command:
“`
docker build -t my-app .
“`
– Replace `my-app` with the desired image name.
– Once the image is built, you can run a container from it using the `docker run` command.
6. Share and collaborate with Docker Hub:
– Docker Hub is a cloud-based registry where you can store and share Docker images.
– Create a Docker Hub account (https://hub.docker.com/) and log in using the Docker CLI:
“`
docker login
“`
– Tag your local image with your Docker Hub username and push it to the registry:
“`
docker tag my-app
docker push
“`
– Replace `
– Now, anyone can pull and run your image from the Docker Hub registry.
This beginner’s guide should give you a good starting point for using Docker. As you gain more experience, you can explore advanced features like Docker Compose for managing multi-container applications, Docker Swarm for orchestration, and Kubernetes for container orchestration at scale.