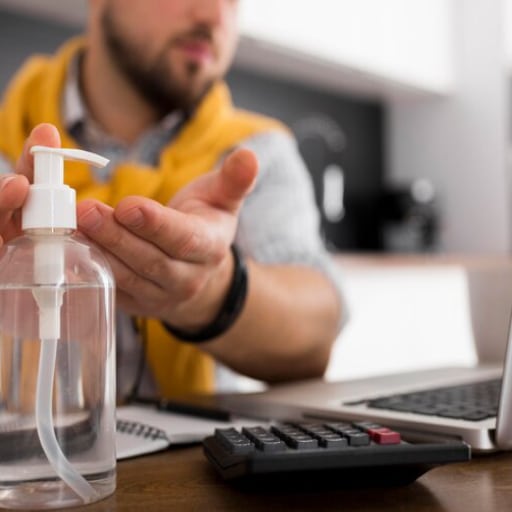
Sure! Here is a step-by-step tutorial on how to dockerize a Flask application:
Step 1: Install Docker
First, make sure you have Docker installed on your machine. You can download and install Docker from the official website (https://www.docker.com/products/docker-desktop).
Step 2: Create a Flask Application
Create a new directory for your Flask application and navigate to it in your terminal. Then, create a new Python virtual environment and activate it:
“`
$ python3 -m venv venv
$ source venv/bin/activate
“`
Next, install Flask using pip:
“`
$ pip install flask
“`
Create a new file called `app.py` and add the following code to it:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def hello():
return ‘Hello, World!’
if __name__ == ‘__main__’:
app.run(debug=True, host=’0.0.0.0′)
“`
Step 3: Create a Dockerfile
In the same directory as your Flask application, create a new file called `Dockerfile` (without any file extension). Open the file and add the following code:
“`
FROM python:3.9-slim-buster
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD [ "python", "app.py" ]
“`
This Dockerfile starts with a base image of Python 3.9, sets the working directory to `/app`, copies the `requirements.txt` file, installs the dependencies, and then copies the entire current directory into the container. Finally, it sets the command to run the `app.py` file.
Step 4: Create a requirements.txt file
In the same directory as your Flask application, create a new file called `requirements.txt` and add the following line to it:
“`
Flask==2.0.1
“`
This file specifies the dependencies required by your Flask application.
Step 5: Build the Docker Image
In your terminal, navigate to the directory containing your Flask application and Dockerfile. Then, build the Docker image using the following command:
“`
$ docker build -t flask-app .
“`
This command builds a Docker image with the tag `flask-app` using the current directory as the build context.
Step 6: Run the Docker Container
Once the Docker image is built, you can run a Docker container based on that image using the following command:
“`
$ docker run -p 5000:5000 flask-app
“`
This command runs a Docker container based on the `flask-app` image and maps port 5000 of the container to port 5000 of the host machine.
Step 7: Test the Flask Application
Open your web browser and navigate to `http://localhost:5000`. You should see the message "Hello, World!" displayed, indicating that your Flask application is running successfully in the Docker container.
That’s it! You have successfully dockerized your Flask application. You can now distribute and deploy your application as a Docker image.