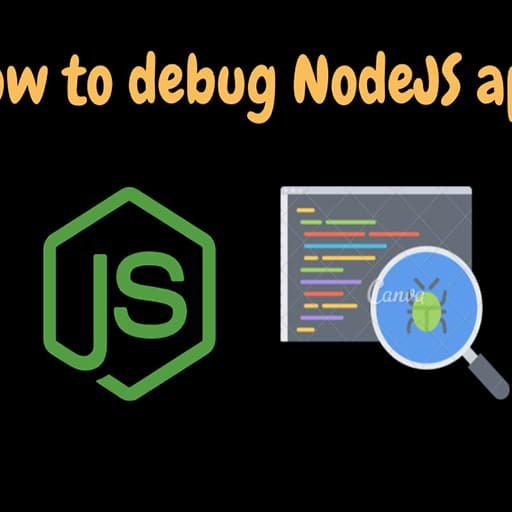
Debugging Node.js applications can sometimes be a challenging task, but with the right tips and tricks, it can become much easier. Here are some useful tips and tricks for debugging Node.js applications:
1. Use the built-in debugger: Node.js comes with a built-in debugger that allows you to set breakpoints, step through code, and inspect variables. You can start the debugger by running your script with the `–inspect` flag, and then connect to it using a Chrome DevTools instance.
2. Use console.log: The simplest way to debug your Node.js application is by using console.log statements. You can print out the values of variables, function calls, and any other information that can help you understand the flow of your code.
3. Use the Node.js debugger module: Node.js provides a debugger module that you can use programmatically in your code. You can set breakpoints, step through code, and inspect variables using this module. To use it, you need to require the `debugger` module and call the `debugger` statement at the point where you want to start debugging.
4. Use a debugger extension: There are several debugger extensions available for popular code editors like Visual Studio Code and Atom. These extensions provide a graphical interface for debugging Node.js applications, making it easier to set breakpoints, step through code, and inspect variables.
5. Use the Node.js inspector API: Node.js provides an inspector API that allows you to programmatically control the debugger. You can use this API to set breakpoints, step through code, and inspect variables. This can be useful if you want to automate the debugging process or integrate it into your testing framework.
6. Use logging libraries: Instead of using console.log statements, you can use logging libraries like Winston or Bunyan. These libraries provide more advanced logging capabilities, such as logging to different output streams, filtering log messages based on severity, and formatting log messages.
7. Use a remote debugger: If your Node.js application is running on a remote server or in a container, you can use a remote debugger to debug it. Remote debuggers allow you to connect to your application from your local machine and debug it as if it were running locally.
8. Use a memory profiler: If your Node.js application is consuming a lot of memory or experiencing memory leaks, you can use a memory profiler to identify the source of the problem. Tools like the Node.js built-in heap profiler or third-party tools like Chrome DevTools can help you analyze memory usage and find memory leaks.
9. Use a code linter: Code linters like ESLint or JSHint can help you catch common coding mistakes and potential bugs before they cause issues in your application. By running a linter on your code, you can identify and fix potential issues early on, reducing the need for debugging.
10. Use unit tests: Writing unit tests for your Node.js application can help you catch bugs early on and make debugging easier. By writing tests that cover different parts of your codebase, you can ensure that your application behaves as expected and quickly identify any regressions or issues.
By following these tips and tricks, you can make the process of debugging Node.js applications more efficient and effective. Remember to always test your code thoroughly and use best practices to minimize the need for debugging in the first place.