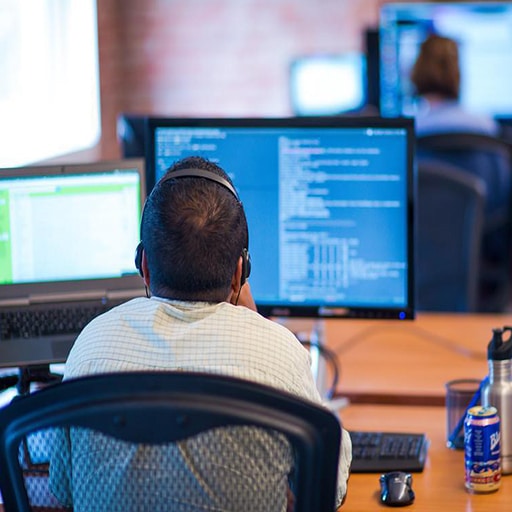
To create dynamic forms with PHP, you can follow these steps:
1. Start by creating an HTML form with the necessary input fields. For example, you can create a form with a text input field and a submit button:
“`html
“`
2. In the `action` attribute of the form, specify the PHP file (`process.php`) that will handle the form submission.
3. In the `process.php` file, you can access the form data using the `$_POST` superglobal variable. For example, to retrieve the value entered in the name field, you can use `$_POST[‘name’]`.
“`php
if ($_SERVER[‘REQUEST_METHOD’] === ‘POST’) {
$name = $_POST[‘name’];
echo "Hello, $name!";
}
?>
“`
4. You can also dynamically generate form fields based on certain conditions or user input. For example, you can add a checkbox field if a specific condition is met:
“`php
if ($_SERVER[‘REQUEST_METHOD’] === ‘POST’) {
$name = $_POST[‘name’];
echo "Hello, $name!";
if ($_POST[‘condition’] === ‘true’) {
echo "
Checkbox is checked!";
}
}
?>
“`
In this example, if the checkbox is checked, the message "Checkbox is checked!" will be displayed along with the name.
5. You can also use loops to generate multiple form fields dynamically. For example, you can use a `foreach` loop to generate a set of radio buttons based on an array of options:
“`php
$options = [‘Option 1’, ‘Option 2’, ‘Option 3’];
if ($_SERVER[‘REQUEST_METHOD’] === ‘POST’) {
$name = $_POST[‘name’];
echo "Hello, $name!";
foreach ($options as $option) {
if ($_POST[‘option’] === $option) {
echo "
Selected option: $option";
}
}
}
?>
“`
In this example, the selected radio button option will be displayed along with the name.
These are just basic examples, and you can customize and extend them based on your specific requirements.