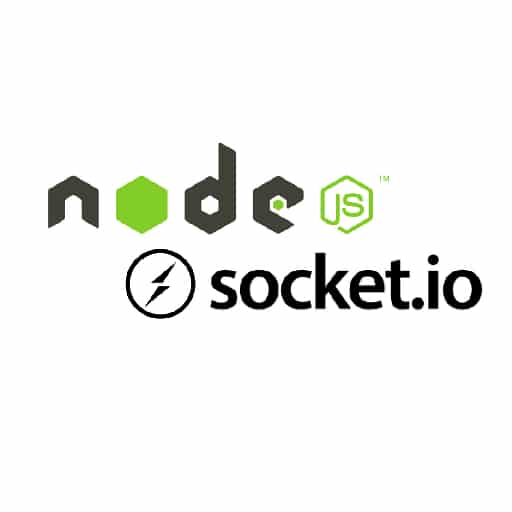
Node.js is a popular runtime environment that allows developers to build scalable and high-performance applications using JavaScript. Socket.io is a library that enables real-time, bidirectional communication between web clients and servers.
By combining Node.js and Socket.io, developers can create real-time applications that can push data from the server to multiple clients instantly. This is particularly useful for applications that require real-time updates, such as chat applications, collaborative tools, and live dashboards.
To build a real-time application with Node.js and Socket.io, follow these steps:
1. Set up a Node.js project: Start by creating a new directory for your project and initializing a new Node.js project using npm. Run the following command in your project directory:
“`
npm init -y
“`
2. Install the required dependencies: Install the `express` and `socket.io` packages using npm. Run the following command:
“`
npm install express socket.io
“`
3. Create a server file: Create a new file called `server.js` and require the necessary modules:
“`javascript
const express = require(‘express’);
const app = express();
const http = require(‘http’).createServer(app);
const io = require(‘socket.io’)(http);
“`
4. Set up the server: Define the server’s behavior and start listening for incoming connections:
“`javascript
app.get(‘/’, (req, res) => {
res.sendFile(__dirname + ‘/index.html’);
});
io.on(‘connection’, (socket) => {
console.log(‘A user connected’);
socket.on(‘disconnect’, () => {
console.log(‘A user disconnected’);
});
});
http.listen(3000, () => {
console.log(‘Server is running on http://localhost:3000’);
});
“`
5. Create a client file: Create a new file called `index.html` and include the Socket.io client library:
“`html
“`
6. Connect to the server: In the client file, establish a connection to the server and handle events:
“`javascript
const socket = io();
socket.on(‘connect’, () => {
console.log(‘Connected to the server’);
});
socket.on(‘disconnect’, () => {
console.log(‘Disconnected from the server’);
});
“`
7. Emit and handle events: Use the `emit` method on the server to send data to connected clients, and use the `on` method on the client to handle incoming events:
“`javascript
// Server
io.on(‘connection’, (socket) => {
socket.emit(‘message’, ‘Welcome to the chat’);
socket.on(‘chat message’, (msg) => {
io.emit(‘chat message’, msg);
});
});
// Client
socket.on(‘message’, (msg) => {
console.log(msg);
});
socket.on(‘chat message’, (msg) => {
console.log(msg);
});
“`
8. Start the server: Run the following command in your project directory to start the server:
“`
node server.js
“`
9. Open the application in a web browser: Open `http://localhost:3000` in multiple browser tabs or windows to see real-time updates in action.
With these steps, you can build a basic real-time application using Node.js and Socket.io. You can further enhance the application by adding features like authentication, private messaging, and real-time notifications.