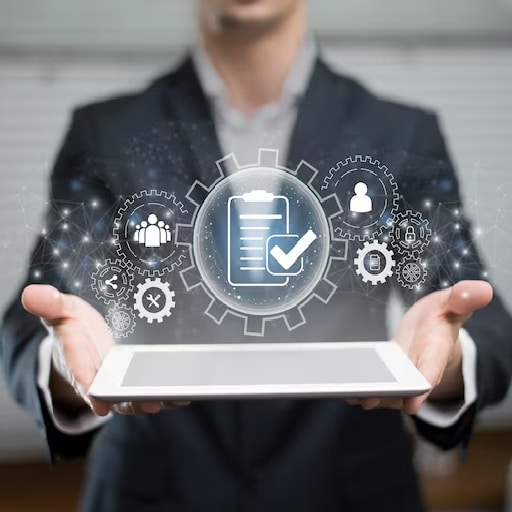
To build a task management system with Ruby on Rails, you can follow these steps:
1. Set up a new Rails application by running the following command in your terminal:
“`
rails new task_management_system
“`
2. Change into the project directory:
“`
cd task_management_system
“`
3. Generate a Task model and migration file:
“`
rails generate model Task title:string description:text completed:boolean
“`
4. Run the database migration to create the tasks table:
“`
rails db:migrate
“`
5. Open the `app/models/task.rb` file and add the following validation for the title:
“`ruby
class Task < ApplicationRecord
validates :title, presence: true
end
“`
6. Generate a Tasks controller:
“`
rails generate controller Tasks
“`
7. Open the `config/routes.rb` file and add the following route for tasks:
“`ruby
Rails.application.routes.draw do
resources :tasks
end
“`
8. Open the `app/controllers/tasks_controller.rb` file and add the following actions:
“`ruby
class TasksController < ApplicationController
before_action :set_task, only: [:show, :edit, :update, :destroy]
def index
@tasks = Task.all
end
def show
end
def new
@task = Task.new
end
def create
@task = Task.new(task_params)
if @task.save
redirect_to @task, notice: ‘Task was successfully created.’
else
render :new
end
end
def edit
end
def update
if @task.update(task_params)
redirect_to @task, notice: ‘Task was successfully updated.’
else
render :edit
end
end
def destroy
@task.destroy
redirect_to tasks_url, notice: ‘Task was successfully destroyed.’
end
private
def set_task
@task = Task.find(params[:id])
end
def task_params
params.require(:task).permit(:title, :description, :completed)
end
end
“`
9. Create the views for tasks by creating the following files:
– `app/views/tasks/index.html.erb`
– `app/views/tasks/show.html.erb`
– `app/views/tasks/new.html.erb`
– `app/views/tasks/edit.html.erb`
In each view file, you can use ERB syntax to display the task information.
10. Start the Rails server:
“`
rails server
“`
11. Open your web browser and visit `http://localhost:3000/tasks` to access the task management system.
That’s it! You now have a basic task management system built with Ruby on Rails. You can further enhance it by adding features like user authentication, task assignment, due dates, etc.